How To Train AI Classification Models
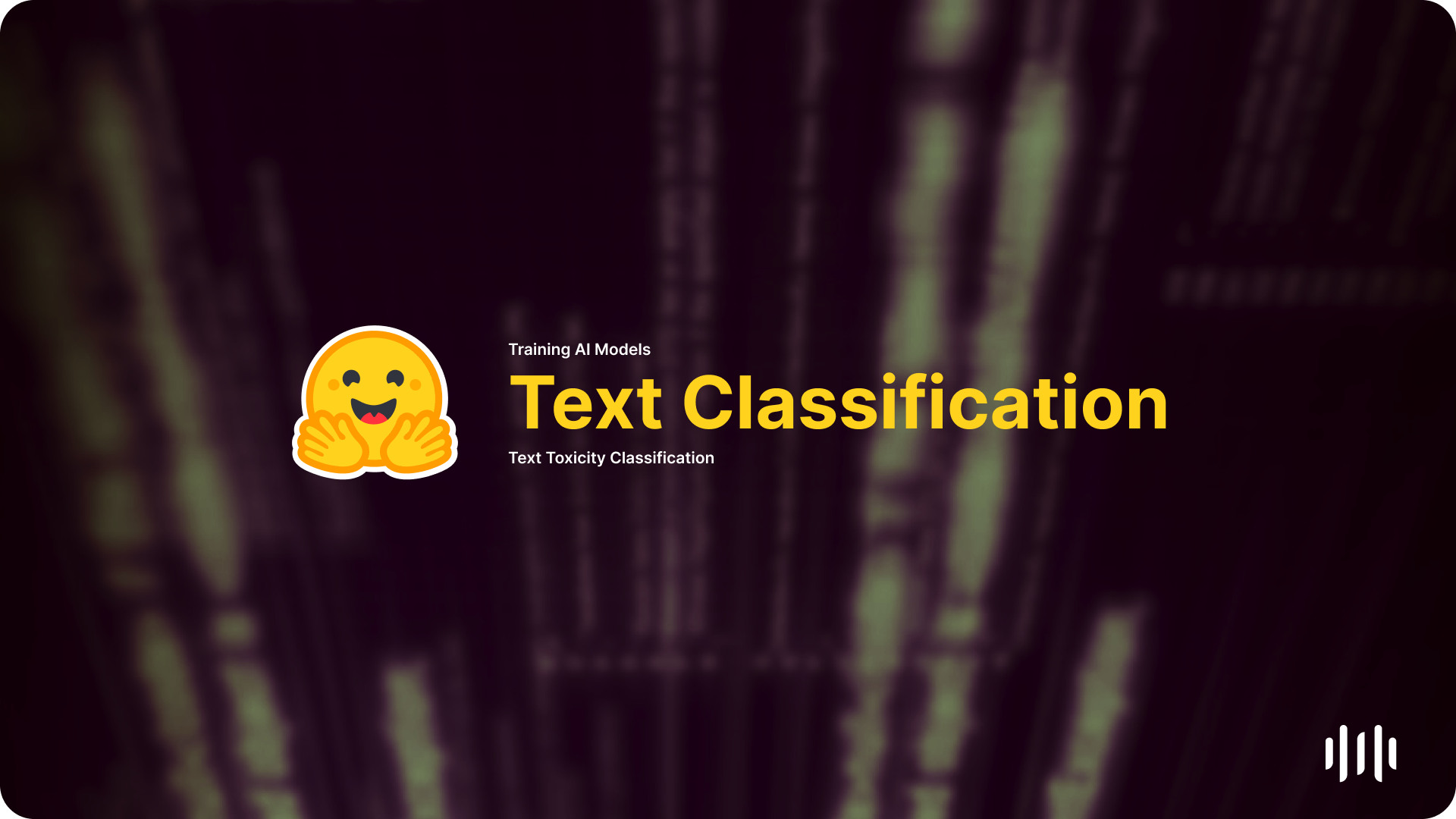
A Text Classification model is an AI-based model trained to categorize or classify text into predefined categories or classes. It learns from a labeled dataset where each text sample is associated with a specific category. The model analyzes a string's patterns, features, and relationships to classify new or unseen text samples. Various applications use Text Classification models, such as sentiment analysis, spam detection, topic classification, and intent recognition. Text Classification models are built using various machine learning algorithms, such as Naive Bayes, Support Vector Machines (SVM), or deep learning algorithms like Recurrent Neural Networks (RNN) or Convolutional Neural Networks (CNN). In this article, we will train a Text Classification model to identify toxic text samples.
Create a Python Virtual Environment
A Python virtual environment is an isolated environment where you can install Python packages without affecting your global Python setup. It's a way to manage dependencies on a per-project basis. This is how we will set up our project:
- Create a directory to store our files. On a Debian-based system we will run:
mkdir AI\ Classifications && cd AI\ Classifications
- Create a virtual environment:
python3 -m venv .env
- Activate your virtual environment: (If you close your terminal, you'll have to re-run this command)
source .env/bin/activate
Install the transformers library
Note that the following is only if you have an NVIDIA GPU. PyTorch and HF Transformers use CUDA to interface to CUDA Cores on your GPU. A Cuda core is much more lightweight than your CPU cores. GPUs can do less advanced arrhythmic, but calculations can be done much faster as you have more cores.
#HF Transformers
pip install git+https://github.com/huggingface/transformers
#PyTorch
pip install torch torchvision torchaudio
### Other libraries for machine learning and data parsing:
pip install pandas datasets scikit-learn accelerate